Overview
vchatCloud sdk
helps to make chat client implementation easy.
Use 'vchatCloud sdk' on Android to use real-time chatting quickly and efficiently.
The quick guide provides brief information about the structure and functions of vchatCloud sdk
,
Each topic provides a guide for application to practical projects based on detailed explanations.
Experience the chat service
Enter chat room
In order to enter the chat room from the 'demo screen', you must include the channel key created in CMS.
If it is before opening the chat room, Link Please learn how to create a chat room and check the channel key.
After that, enter the nickname and emoji to be used in the chat room and press the OK button to enter the chat room.
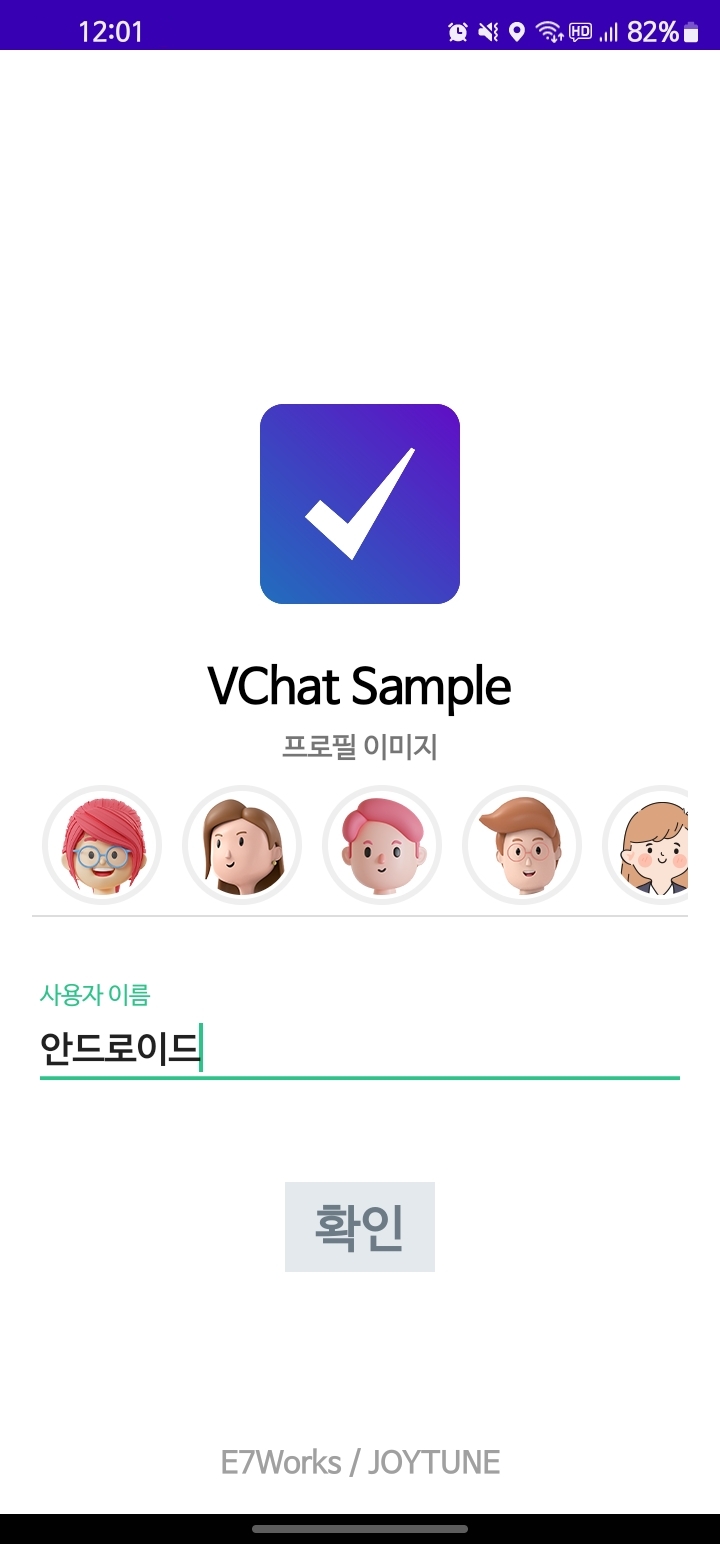
chat
For chat, enter the message to be sent in the text input box and press the send button or press the enter key to send.
If message transmission is proceeding normally, try entering the chat room with another device or PC.
When entering as another user, enter the same channel key to enter the same chat room and send and receive chat messages.
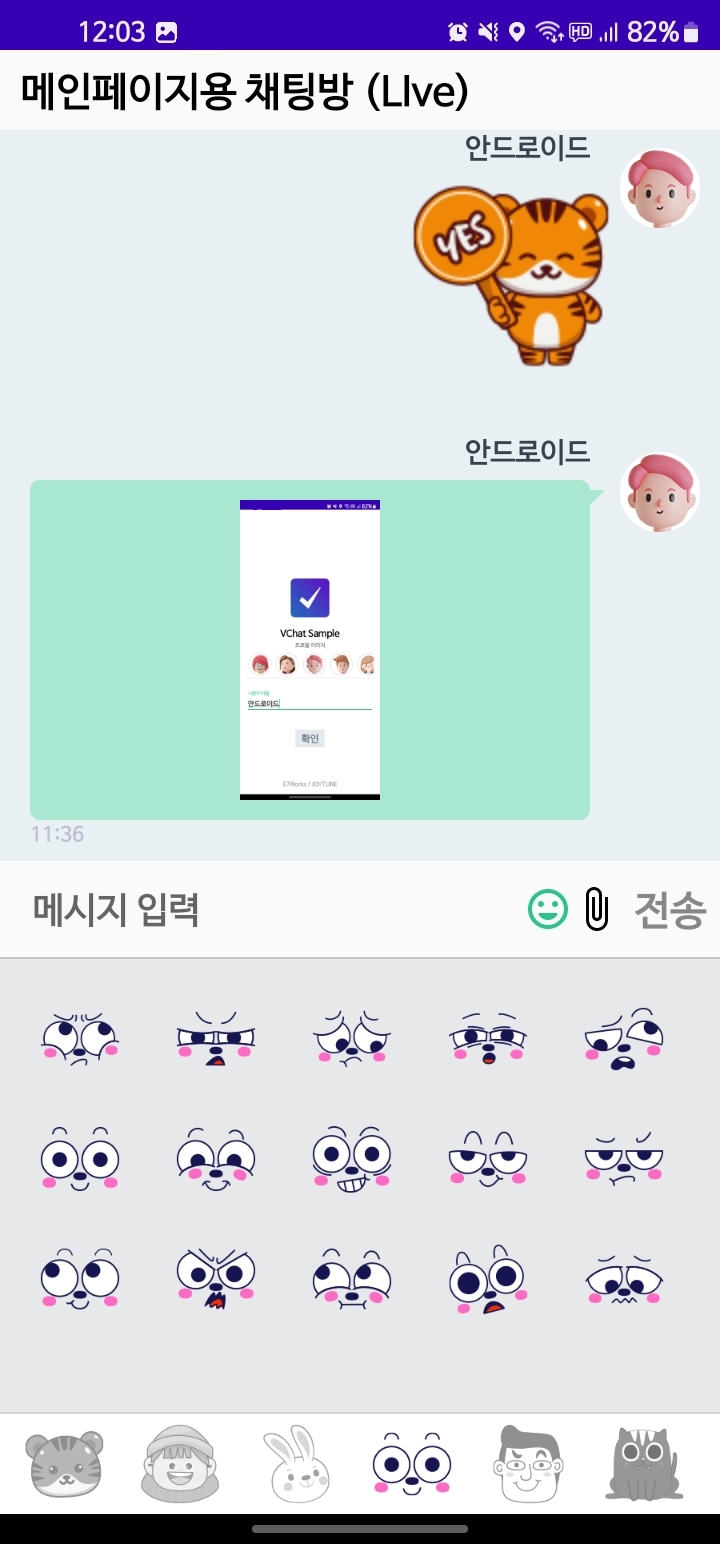
Check implementation code
Enter chat room
@Override
protected void onStart() {
private static Channel channel = VChatCloud.getInstance().joinChannel(options, new JoinChannelCallback() {
public void callback(JSONArray history, VChatCloudException e) {
super. callback(history, e);
int historySize = history. size() -1;
for (; historySize >= 0; historySize--) {
messageExposure(new Message((JSONObject) history.get(historySize)), false);
}
$Title.setText(channel.getRoomName());
PreferenceManager.setString(getApplicationContext(),"nickName", nick_name);
}
});
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
Channel object event handling
send messages
channel.sendMessage(param, new ChannelCallback() {
@Override
public void callback(Object o, VChatCloudException e) {
if (e != null) {
}
$RecyclerView.smoothScrollToPosition($Adapter.getItemCount());
}
});
1
2
3
4
5
6
7
8
9
10
Send a message to a specific user in the chat room
channel.sendWhisper(json, new ChannelCallback() {
@Override
public void callback(Object o, VChatCloudException e) {
if (e != null) {
}
$RecyclerView.smoothScrollToPosition($Adapter.getItemCount());
}
});
1
2
3
4
5
6
7
8
9
10
An event that occurs when a message is posted in the chat room
public void onNotifyMessage(JSONObject data) {
System.err.println(data);
Message msg = new Message(data);
msg.setType("msg");
messageExposure(msg, false);
}
1
2
3
4
5
6
7
An event that occurs when a whisper message is uploaded to a connected user
public void onPersonalWhisper(JSONObject data) {
Message msg = new Message(data);
msg.setType("preWhisper");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when a notification message is posted in the chat room
public void onNotifyNotice(JSONObject data) {
Message msg = new Message(data);
msg.setType("notice");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when a custom message is posted in the chat room
public void onNotifyCustom(JSONObject data) {
Message msg = new Message(data);
msg.setType("custom");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when a new user accesses the chat room
public void onNotifyJoinUser(JSONObject data) {
Message msg = new Message(data);
if (!nick_name.equalsIgnoreCase(msg.getNickName())) {
msg.setType("join");
msg.setMessage(msg.getNickName() + " has entered.");
messageExposure(msg, false);
}
}
1
2
3
4
5
6
7
8
An event that occurs when a user leaves the chat room
public void onNotifyLeaveUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("leave");
msg.setMessage(msg.getNickName() + " has left.");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when you are kicked out of the chat room
public void onNotifyKickUser(JSONObject data) {
Message msg = new Message(data);
msg. setType("kick");
messageExposure(msg, false);
}
1
2
3
4
5
6
Event that occurs when banishment is lifted in the chat room
public void onNotifyUnkickUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("unKick");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when a user is restricted from writing in a chat room
public void onNotifyMuteUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("mute");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when a user's writing restriction is lifted in a chat room
public void onNotifyUnmuteUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("unMute");
messageExposure(msg, false);
}
1
2
3
4
5
6
Notification to existing logged-in users in case of duplicate login attempts
public void onPersonalDuplicateUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("duplicate");
messageExposure(msg, false);
}
1
2
3
4
5
6
An event that occurs when writing is restricted in a chat room
public void onPersonalMuteUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("preMute");
messageExposure(msg, false);
}
1
2
3
4
5
6
Event that occurs when the writing restriction is lifted in the chat room
public void onPersonalUnmuteUser(JSONObject data) {
Message msg = new Message(data);
msg.setType("perUnMute");
messageExposure(msg, false);
}
1
2
3
4
5
6