# How to use webRTC
To use webRTC, vchatCloud and channel object declarations are required.
Before reading this document, please read the contents of Preparation
before proceeding.
# webRTC Workflow
A webRTC workflow in vchatCloud.
If you check the picture below, you can more easily understand how webRTC works and what code you need to write.
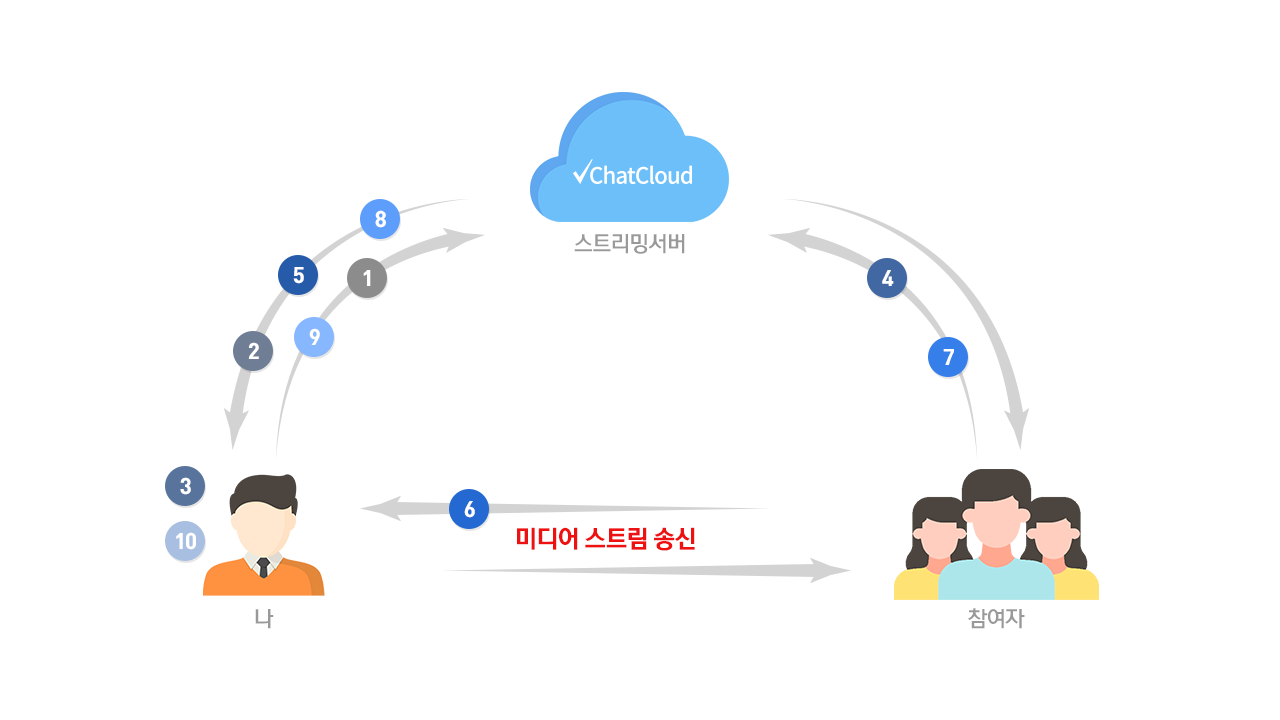
- I enter the video chat room.
- Connect to the server using the
vChatCloud.joinChannel({ roomId, clientKey, nickName }, callback)
function
or thejoinRoom(roomId, clientKey, nickName, callback)
function defined in the example code.
- Connect to the server using the
- My entry event occurs.
- I enter (local media source addition event) occurs,
and you can write the necessary logic (video tag and stream setting, etc.).
- I enter (local media source addition event) occurs,
- My microphone and camera ON/OFF event occurs.
- My camera on/off event, My microphone on/off event occurs and
You can write the necessary logic (set an alternative image when no video is output, display a mute icon, etc.).
- My camera on/off event, My microphone on/off event occurs and
- The participant enters the server.
- A participant entry event occurs.
- Participant entry (remote media source addition event) occurs and
You can write the necessary logic (such as creating video tags for participants and setting up streams).
- Participant entry (remote media source addition event) occurs and
- The participant's microphone and camera ON/OFF event occurs.
- Participant camera ON/OFF event, Participant microphone ON/OFF event occurs and
You can write the necessary logic (set an alternative image when no video is output, display a mute icon, etc.).
- Participant camera ON/OFF event, Participant microphone ON/OFF event occurs and
- The participant leaves.
- Participant exit event occurs.
- Participant exit (remote connection termination event) occurs and
You can write the necessary logic (deleting the video tag of an absent participant, etc.).
- Participant exit (remote connection termination event) occurs and
- I leave the video chat room.
- Use the
vChatCloud.disconnect()
function or theexit(p)
function defined in the example code to
terminate the connection with the server in the video chat room.
- Use the
- I exit event occurs.
- I Exit (Local Media Source Remove Event) occurs and
You can write the necessary logic (deleting the video tag of an absent participant, etc.).
- I Exit (Local Media Source Remove Event) occurs and
# Event type list
You can move to the corresponding section by clicking on the text.
- I enter (local media source addition event):
rtcLocalStreamAppend
- I Exit (Local Media Source Remove Event):
rtcLocalStreamRemove
- Participant entry (remote media source addition event):
rtcRemoteStreamAppend
- Participant exit (remote connection termination event):
rtcRemoteStreamRemove
- My camera on/off event:
rtcLocalVideoChanged
- My microphone on/off event:
rtcLocalAudioChanged
- Participant camera ON/OFF event:
rtcRemoteVideoChanged
- Participant microphone ON/OFF event:
rtcRemoteAudioChanged
# I enter (local media source addition event)
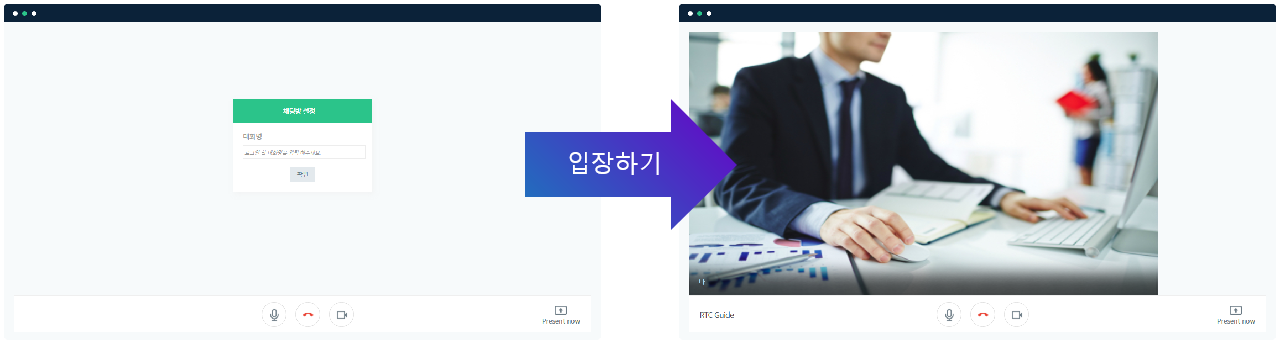
This event occurs when I first enter the chat room.
When this event occurs, you need to set the media stream to the srcObject
of the video
tag so that you can see your own media source.
In the example code below, Create a video
tag to output my video,
and set the target(MediaStream)
value received from event
in the srcObject
property of the tag.
After that, the autoplay function of video
was turned on and the video
tag was inserted and outputted at the displayed position.
If you want to check the operation, just click Run Pen
below.
After executing, you can edit the code and check the reflected result immediately.
If you want to start over, click Rerun
at the bottom right.
View code details
function videoInit() {
channel.on("rtcLocalStreamAppend", function (event) {
// I enter event occured
myVideo = document.createElement("video"); // create video tag
let stream = event.target; // Get MediaStream information from event.target
myVideo.srcObject = stream; // Set MediaStream information in the srcObject property of video
myVideo.setAttribute("autoplay", true); // Set autoplay on video (default starts from stopped)
myCam.append(myVideo); // Insert video into myCam
});
}
let myCam;
window.addEventListener("load", function () {
...
// When you click the Register button after entering your nickname
$('.login').submit(function (e) {
myCam = $(".my_cam");
...
joinRoom(
channelKey, // Key value issued by CMS
clientKey, // User's unique key value
userNick, // user nickname
// Callback function to be executed after channel creation
function (err, history) {
if (err) {
openError(err.code, function () {
vChatCloud.disconnect(); // Connection terminated due to an error
});
} else {
videoInit(); // videoInit(); Execution
}
}
);
});
});
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
Currently, if you press the camera off button, the screen freezes, and it is difficult to check even if you press the microphone off button.
It's because you haven't written the logic for that part yet. Related content will be dealt with in My camera on/off event.
Also, currently, there are multiple video areas when reconnecting after termination, which will be dealt with in I Exit (Local Media Source Remove Event).
# Event data
{
"type": "rtcLocalStreamAppend",
"target": {...},
"clientKey": "xxxxxxxx",
"client": {
"clientKey": "xxxxxxxx",
"nickName": "e7works",
"grade": "user"
}
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | MediaStream | Added media stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
client | Object | Information about the connected device (information about clientKey , nickName , grade ) |
# I Exit (Local Media Source Remove Event)
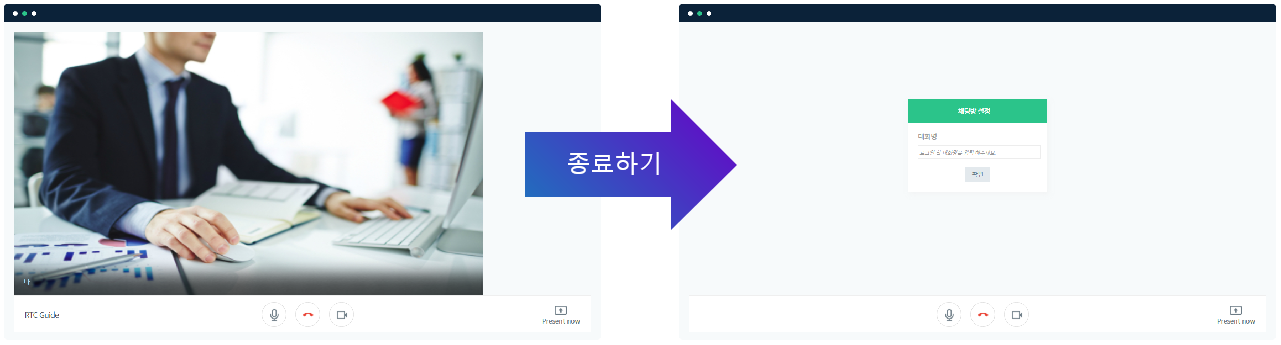
This event occurs when I disconnect from the chat room.
After closing the connection, you can initialize the chat room or write the necessary logic, such as moving to another page.
In the example code below, the chat room is initialized and the login screen is displayed again.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam > video'); // A tag that encloses a video tag that displays me.
html.remove(); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"type": "rtcLocalStreamRemove",
"target": null,
"clientKey": "xxxxxxxxx",
"client": {
"clientKey": "xxxxxxxxx",
"nickName": "e7works",
"grade": "user"
}
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | MediaStream | Added media stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
client | Object | Information about the connected device (information about clientKey , nickName , grade ) |
# Participant entry (remote media source addition event)
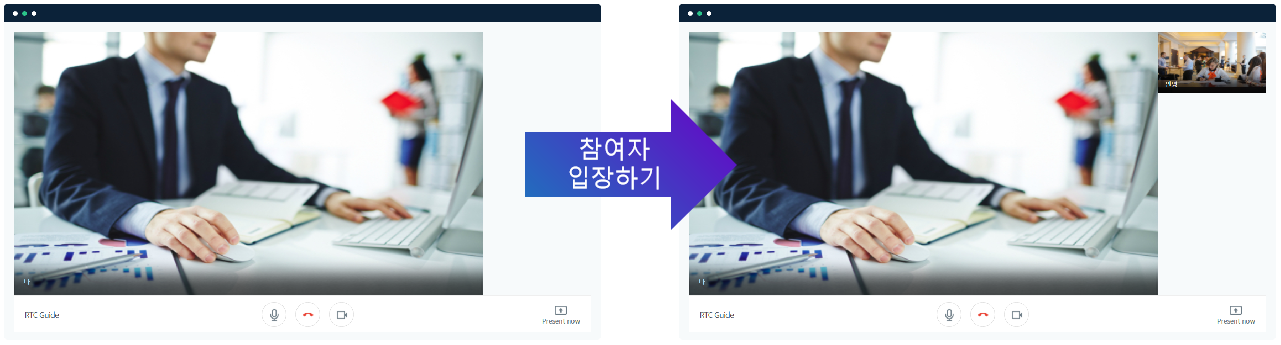
This event occurs when a participant connects to the chat room.
From this point on, you can video chat with other users as well as the user directly connected to the chat room.
When the event occurs, the media stream must be set in the srcObject
of the video
tag so that the media source of the external visitor can be viewed.
In the example code, the participant's video is displayed next to my video in a small size and the number of people.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam > video'); // A tag that encloses a video tag that displays me.
html.remove(); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
Currently, when the remote user presses the camera off button, the screen freezes, and the reason it is difficult to check even
if the remote user presses the microphone off button is because the logic for that part has not been written yet.
Related content will be dealt with in Participant camera ON/OFF event.
Also, currently, the video area does not disappear when the remote user terminates the connection,
which will be dealt with in Participant exit (remote connection termination event).
# Event data
{
"type": "rtcRemoteStreamAppend",
"target": {...},
"clientKey": "xxxxxxxx",
"client": {
"clientKey": "xxxxxxxx",
"nickName": "e7works",
"grade": "user"
}
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | MediaStream | Added media stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
client | Object | Information about the connected device (information about clientKey , nickName , grade ) |
# Participant exit (remote connection termination event)
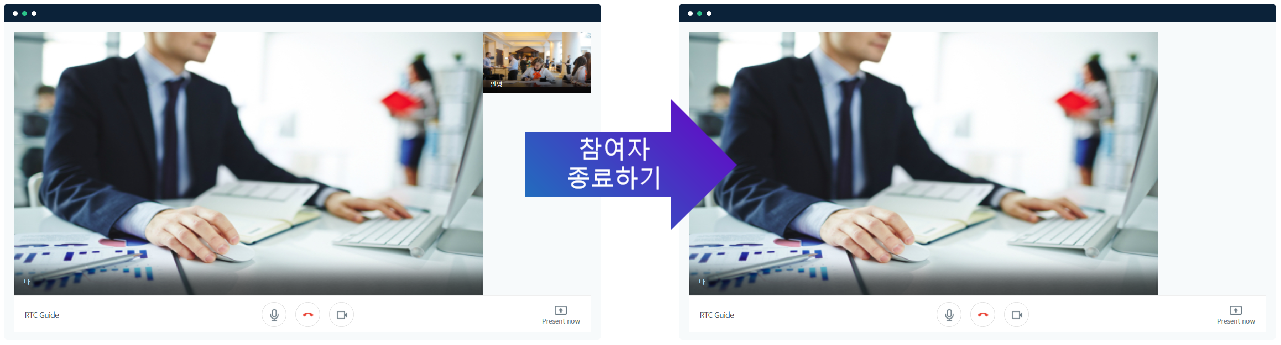
This event occurs when a participant terminates the connection.
After the participant's connection is terminated, you can write the necessary logic,
such as deleting the video
tag that outputs the media stream of the user or the div
tag wrapped around it.
In the example code, the tag surrounding the remote user's video
was deleted and disappeared again.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// Participant connection termination event
channel.on('rtcRemoteStreamRemove', function(event) {
let html = $(`.remote_cam_wrap div[name=${event.clientKey}]`); // A tag surrounding the video tag that displays the remote accessor.
if (html.length) {
html.remove(); // Delete tags (disabled)
}
});
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam'); // A tag that encloses a video tag that displays me.
html.html(''); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"client": undefined,
"type": "rtcRemoteStreamRemove",
"target": null,
"clientKey": "xxxxxxxx"
}
2
3
4
5
6
- Event value
Value | Type | Described |
---|---|---|
client | undefined | - |
type | String | Event type that occurred |
target | null | - |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
# My camera on/off event
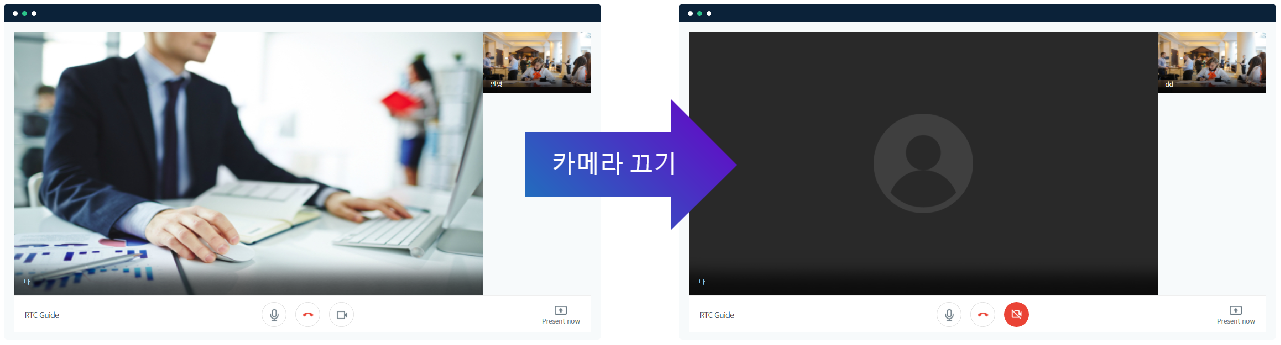
This event occurs when the video source is changed (camera ON/OFF) in my media stream.
When the corresponding event occurs, in accordance with the status of the media stream, when the camera is turned off,
an alternate image should be jumped to avoid seeing the frozen screen.
The example code hides the video and displays an alternate image when I turn off the camera.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// my video source change event
channel.on('rtcLocalVideoChanged', function(event) {
let is_cam = event.enable; // check my camera status
$('.my_cam video').css('display', is_cam ? '' : 'none'); // Hide video tag when my camera is disabled
$('.my_cam .camera_off').css('display', !is_cam ? 'block' : 'none'); // Show alternate image when my camera is disabled
// Set the camera on/off button state.
$('.cam-footer .cam-btn .cam')
.toggleClass('btn_on', is_cam)
.toggleClass('btn_off', !is_cam);
// Set whether to show on_cam.png or off_cam.png according to is_cam status.
$('.cam-footer .cam-btn .cam img').attr(
'src',
is_cam
? '/img/webRTC/on_cam.png' // camera on icon address
: '/img/webRTC/off_cam.png' // camera off icon address
);
});
// Participant connection termination event
channel.on('rtcRemoteStreamRemove', function(event) {
let html = $(`.remote_cam_wrap div[name=${event.clientKey}]`); // A tag surrounding the video tag that displays the remote accessor.
if (html.length) {
html.remove(); // Delete tags (disabled)
}
});
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam'); // A tag that encloses a video tag that displays me.
html.html(''); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"type": "rtcLocalVideoChanged",
"target": {
"kind": "video", // Camera: video, Microphone: audio
...
},
"stream": {},
"clientKey": "xxxxxxxx",
"enable": true,
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | MediaStreamTrack | Source where the event occurred (camera: video , microphone: audio ) |
stream | MediaStream | Live video stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
enable | Boolean | Whether to activate after an event occurs ( On: true , Off: false ) |
# My microphone on/off event
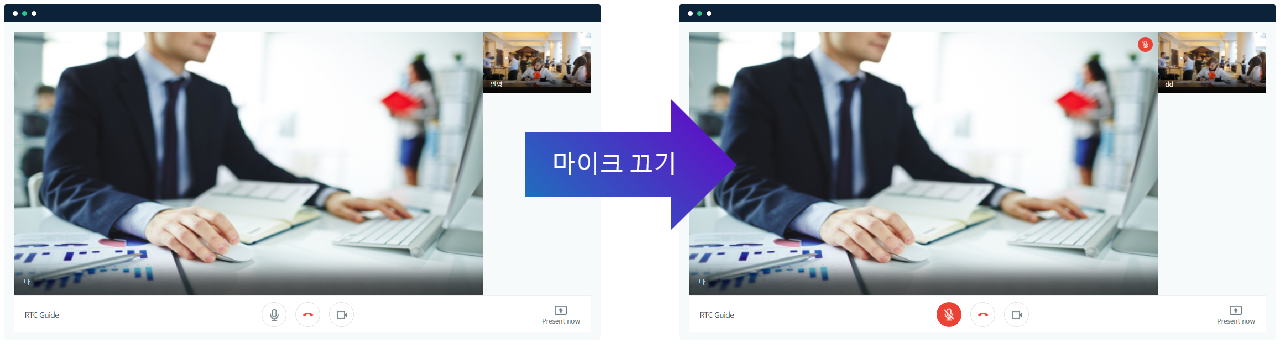
This event is fired when my media stream has changed (microphone ON/OFF).
When that event occurs, we need to make it easy to know when the microphone is off in accordance with the state of the media stream.
In the example code, a mute image is displayed on the top right of the screen when the microphone is off.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// my microphone state change event
channel.on('rtcLocalAudioChanged', function(event) {
let is_mic = event.enable;
$('.mic_off').css('display', !is_mic ? 'block' : 'none');
$('.cam-footer .cam-btn .mic')
.toggleClass('btn_on', is_mic)
.toggleClass('btn_off', !is_mic);
$('.cam-footer .cam-btn .mic img').attr(
'src',
is_mic
? '/img/webRTC/on_mic.png' // Microphone ON image address
: '/img/webRTC/off_mic.png' // Microphone OFF image address
);
});
// my video source change event
channel.on('rtcLocalVideoChanged', function(event) {
let is_cam = event.enable; // check my camera status
$('.my_cam video').css('display', is_cam ? '' : 'none'); // Hide video tag when my camera is disabled
$('.my_cam .camera_off').css('display', !is_cam ? 'block' : 'none'); // Show alternate image when my camera is disabled
// Set the camera on/off button state.
$('.cam-footer .cam-btn .cam')
.toggleClass('btn_on', is_cam)
.toggleClass('btn_off', !is_cam);
// Set whether to show on_cam.png or off_cam.png according to is_cam status.
$('.cam-footer .cam-btn .cam img').attr(
'src',
is_cam
? '/img/webRTC/on_cam.png' // camera on icon address
: '/img/webRTC/off_cam.png' // camera off icon address
);
});
// Participant connection termination event
channel.on('rtcRemoteStreamRemove', function(event) {
let html = $(`.remote_cam_wrap div[name=${event.clientKey}]`); // A tag surrounding the video tag that displays the remote accessor.
if (html.length) {
html.remove(); // Delete tags (disabled)
}
});
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam'); // A tag that encloses a video tag that displays me.
html.html(''); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"type": "rtcLocalAudioChanged",
"target": {
"kind": "audio", // Camera: video, Microphone: audio
...
},
"stream": {},
"clientKey": "xxxxxxxx",
"enable": false
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | Object | Source where the event occurred (camera: video , microphone: audio ) |
stream | MediaStream | Live video stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
enable | Boolean | Whether to activate after an event occurs ( On: true , Off: false ) |
# Participant camera ON/OFF event
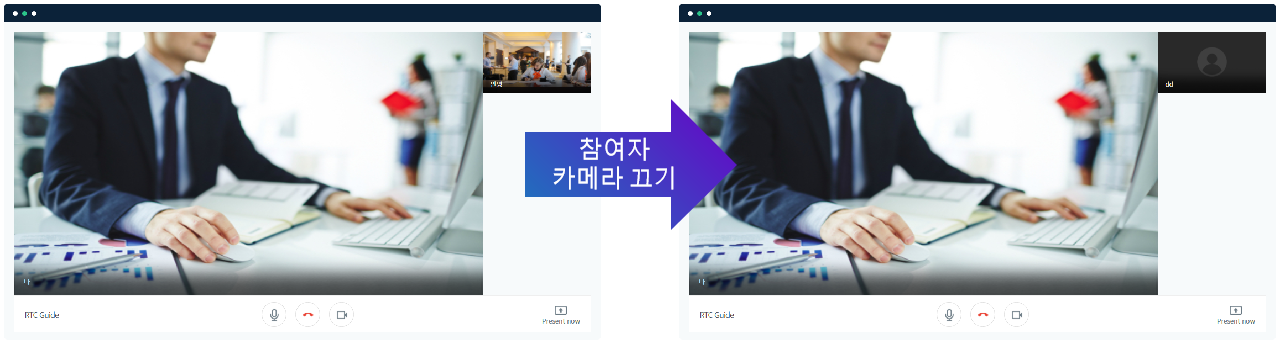
This event occurs when the video source is changed in the participant's media stream (camera ON/OFF, screen sharing mode, etc.).
When the corresponding event occurs, in accordance with the status of the media stream, when the camera is turned off,
an alternate image should be jumped to avoid seeing the frozen screen.
The example code hides the video and displays an alternate image when the participant turns off the camera.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// Participant camera state change event
channel.on('rtcRemoteVideoChanged', function(event) {
let is_cam = event.enable; // Check participant's camera status
$(`.remote_cam[name=${event.clientKey}] video`).css('display', is_cam ? '' : 'none'); // Hide video tag when participant's camera is inactive
$(`.remote_cam[name=${event.clientKey}] .camera_off`).css('display', !is_cam ? 'block' : 'none'); // Show alternate image when participant's camera is inactive
});
// my mic state change event
channel.on('rtcLocalAudioChanged', function(event) {
let is_mic = event.enable;
$('.mic_off').css('display', !is_mic ? 'block' : 'none');
$('.cam-footer .cam-btn .mic')
.toggleClass('btn_on', is_mic)
.toggleClass('btn_off', !is_mic);
$('.cam-footer .cam-btn .mic img').attr(
'src',
is_mic
? '/img/webRTC/on_mic.png' // Microphone ON image address
: '/img/webRTC/off_mic.png' // Microphone OFF image address
);
});
// my video source change event
channel.on('rtcLocalVideoChanged', function(event) {
let is_cam = event.enable; // check my camera status
$('.my_cam video').css('display', is_cam ? '' : 'none'); // Hide video tag when my camera is disabled
$('.my_cam .camera_off').css('display', !is_cam ? 'block' : 'none'); // Show alternate image when my camera is disabled
// Set the camera on/off button state.
$('.cam-footer .cam-btn .cam')
.toggleClass('btn_on', is_cam)
.toggleClass('btn_off', !is_cam);
// Set whether to show on_cam.png or off_cam.png according to is_cam status.
$('.cam-footer .cam-btn .cam img').attr(
'src',
is_cam
? '/img/webRTC/on_cam.png' // camera on icon address
: '/img/webRTC/off_cam.png' // camera off icon address
);
});
// Participant connection termination event
channel.on('rtcRemoteStreamRemove', function(event) {
let html = $(`.remote_cam_wrap div[name=${event.clientKey}]`); // A tag surrounding the video tag that displays the remote accessor.
if (html.length) {
html.remove(); // Delete tags (disabled)
}
});
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam'); // A tag that encloses a video tag that displays me.
html.html(''); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"type": "rtcRemoteVideoChanged",
"target": {
"kind": "video", // Camera: video, Microphone: audio
...
},
"stream": {},
"clientKey": "xxxxxxxx",
"enable": true,
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | MediaStreamTrack | Source where the event occurred (camera: video , microphone: audio ) |
stream | MediaStream | Live video stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
enable | Boolean | Whether to activate after an event occurs ( On: true , Off: false ) |
# Participant microphone ON/OFF event
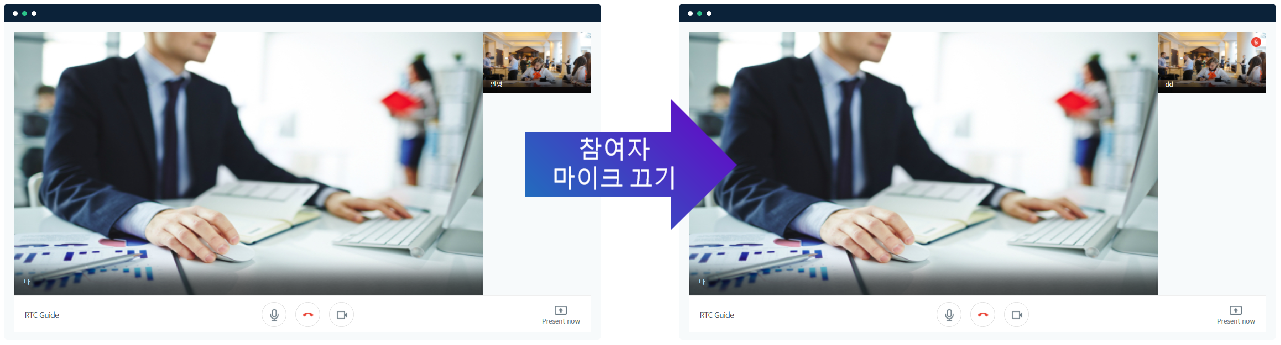
This event is raised when the participant's media stream changes (microphone ON/OFF).
When that event occurs, we need to make it easy to know when the microphone is off in line with the state of the media stream.
In the example code, a mute image is displayed on the top right of the screen when the microphone is off.
If you want to check the operation, just click Run Pen
below.
View code details
function videoInit() {
// Participant microphone state change event
channel.on('rtcRemoteAudioChanged', function(event) {
let is_mic = event.enable;
$(`.remote_cam[name=${event.clientKey}] .mic_off`).css('display', !is_mic ? 'block' : 'none'); // Show/hide mute icon on participant's microphone status
});
// Participant camera state change event
channel.on('rtcRemoteVideoChanged', function(event) {
let is_cam = event.enable; // Check participant's camera status
$(`.remote_cam[name=${event.clientKey}] video`).css('display', is_cam ? '' : 'none'); // Hide video tag when participant's camera is inactive
$(`.remote_cam[name=${event.clientKey}] .camera_off`).css('display', !is_cam ? 'block' : 'none'); // Show alternate image when participant's camera is inactive
});
// my mic state change event
channel.on('rtcLocalAudioChanged', function(event) {
let is_mic = event.enable;
$('.mic_off').css('display', !is_mic ? 'block' : 'none');
$('.cam-footer .cam-btn .mic')
.toggleClass('btn_on', is_mic)
.toggleClass('btn_off', !is_mic);
$('.cam-footer .cam-btn .mic img').attr(
'src',
is_mic
? '/img/webRTC/on_mic.png' // Microphone ON image address
: '/img/webRTC/off_mic.png' // Microphone OFF image address
);
});
// my video source change event
channel.on('rtcLocalVideoChanged', function(event) {
let is_cam = event.enable; // check my camera status
$('.my_cam video').css('display', is_cam ? '' : 'none'); // Hide video tag when my camera is disabled
$('.my_cam .camera_off').css('display', !is_cam ? 'block' : 'none'); // Show alternate image when my camera is disabled
// Set the camera on/off button state.
$('.cam-footer .cam-btn .cam')
.toggleClass('btn_on', is_cam)
.toggleClass('btn_off', !is_cam);
// Set whether to show on_cam.png or off_cam.png according to is_cam status.
$('.cam-footer .cam-btn .cam img').attr(
'src',
is_cam
? '/img/webRTC/on_cam.png' // camera on icon address
: '/img/webRTC/off_cam.png' // camera off icon address
);
});
// Participant connection termination event
channel.on('rtcRemoteStreamRemove', function(event) {
let html = $(`.remote_cam_wrap div[name=${event.clientKey}]`); // A tag surrounding the video tag that displays the remote accessor.
if (html.length) {
html.remove(); // Delete tags (disabled)
}
});
// participant connection event
channel.on('rtcRemoteStreamAppend', function(event) {
let stream = event.target;
// Create a new div using the clientKey data received from the event
let html = $(`<div>`, {
name: event.clientKey,
class: 'remote_cam',
});
remoteCamList.append(html); // Insert the HTML source into the wrapping tag
let remoteVideo = document.createElement('video');
remoteVideo.srcObject = stream; // Set media source to HTMLVideoElement
remoteVideo.setAttribute('autoplay', true); // Set auto play
$(html).append(remoteVideo);
let userNick = $('<p>').text(event.client.nickName);
$(html).append(userNick); // Set the participant's nickname
$('.nocam').toggleClass('active', stream.getVideoTracks().length == 0); // Change the screen according to the status of the camera
$('.nomic').toggleClass('active', stream.getAudioTracks().length == 0); // Change the screen according to the state of the microphone
});
// when I get disconnected
channel.on('rtcLocalStreamRemove', function(event) {
let html = $('.my_cam'); // A tag that encloses a video tag that displays me.
html.html(''); // Delete tags (delete non-working video tags)
});
// when I connect
channel.on('rtcLocalStreamAppend', function(event) {
myVideo = document.createElement('video');
let stream = event.target;
myVideo.srcObject = stream;
myVideo.setAttribute('autoplay', true);
myCam.append(myVideo);
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
Precautions! After execution, if you do not disconnect by clicking the quit button below,
your camera and microphone can be shared until the page is moved or closed.
# Event data
{
"type": "rtcRemoteAudioChanged",
"target": {
"kind": "audio", // Camera: video, Microphone: audio
...
},
"stream": {},
"clientKey": "xxxxxxxx",
"enable": false
}
2
3
4
5
6
7
8
9
10
- Event value
Value | Type | Described |
---|---|---|
type | String | Event type that occurred |
target | Object | Source where the event occurred (camera: video , microphone: audio ) |
stream | MediaStream | Live video stream |
clientKey | String | Connection device setting unique key (required to identify one of several participants) |
enable | Boolean | Whether to activate after an event occurs ( On: true , Off: false ) |