# Quick Guide
# STEP1. Sign up for free
Go to the first page of vchatCloud and click the Free Use button (opens new window) at the top
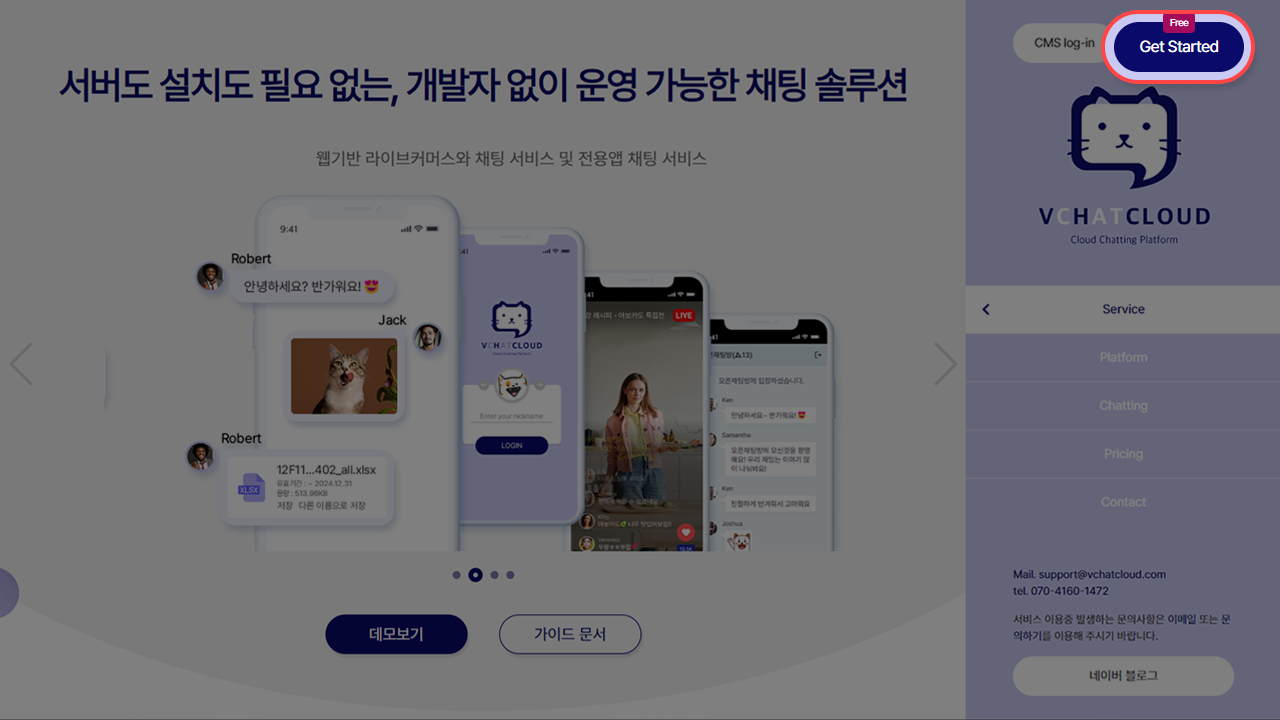
In the Sign up form, email address (actual email address where you can receive authentication email)
, name, password
and enter verify password and accept the terms and conditions.
Please complete the membership registration.
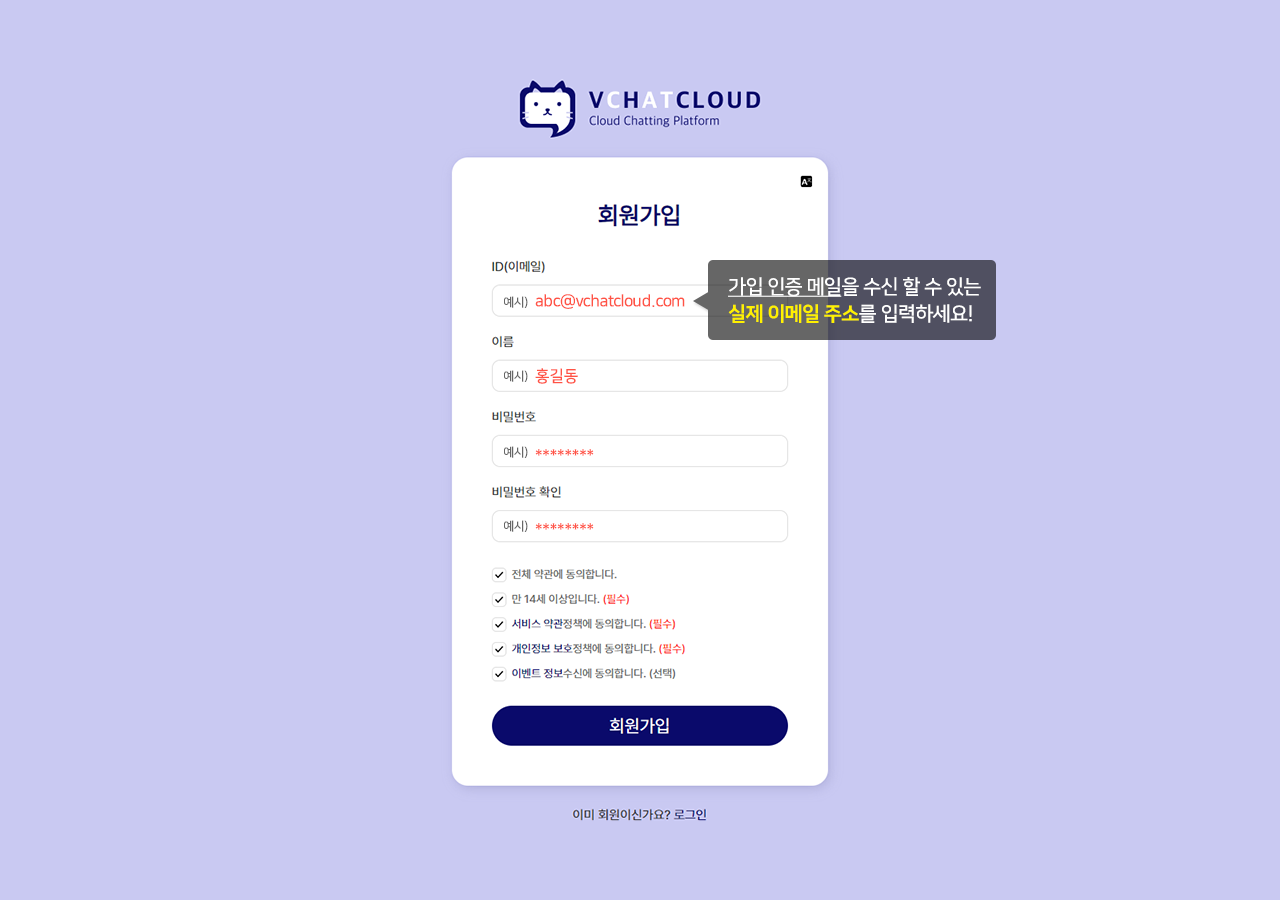
A confirmation email will be sent to the registered email address.
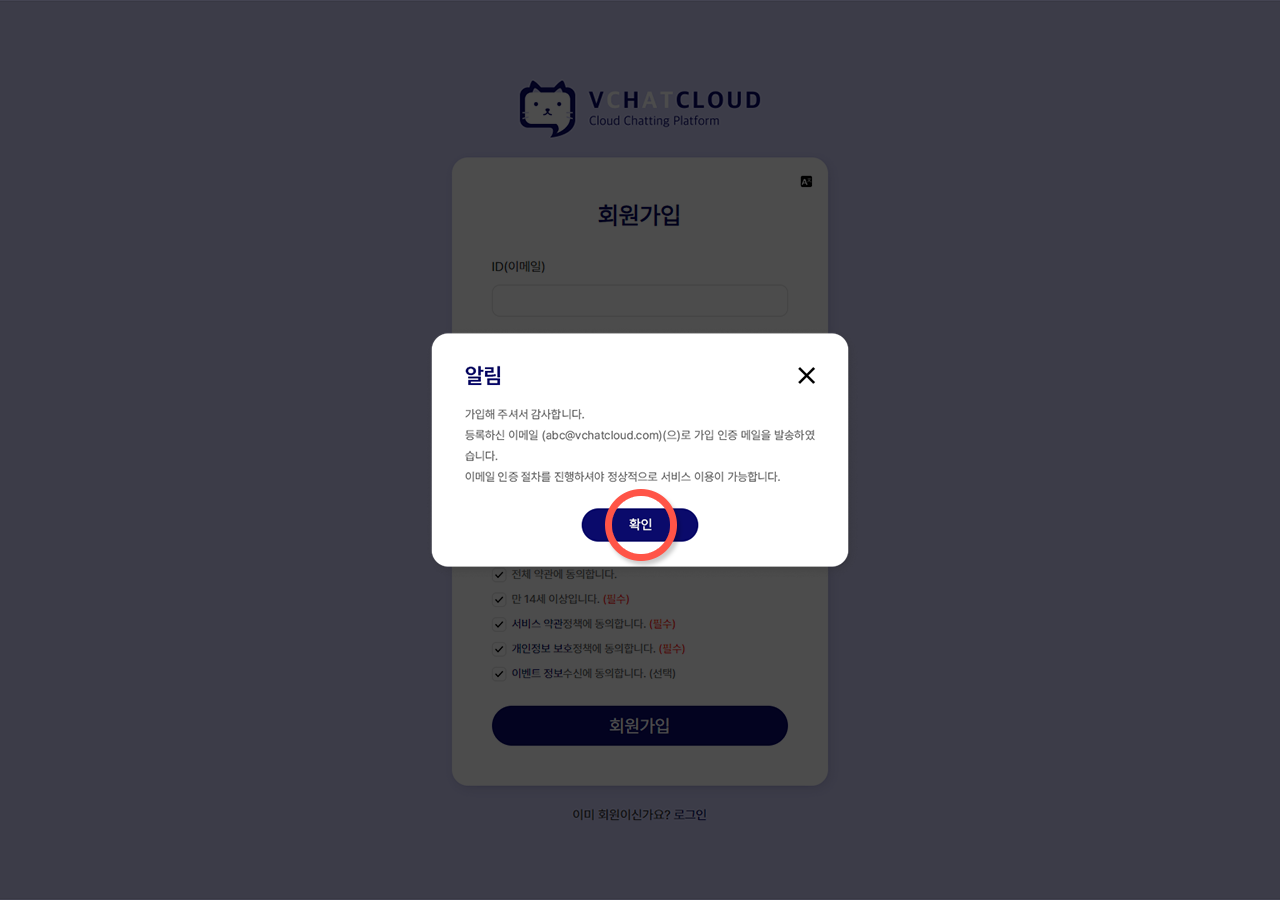
Register as a member by clicking the "here" link in the body of the verification confirmation email The Membership registration is complete.
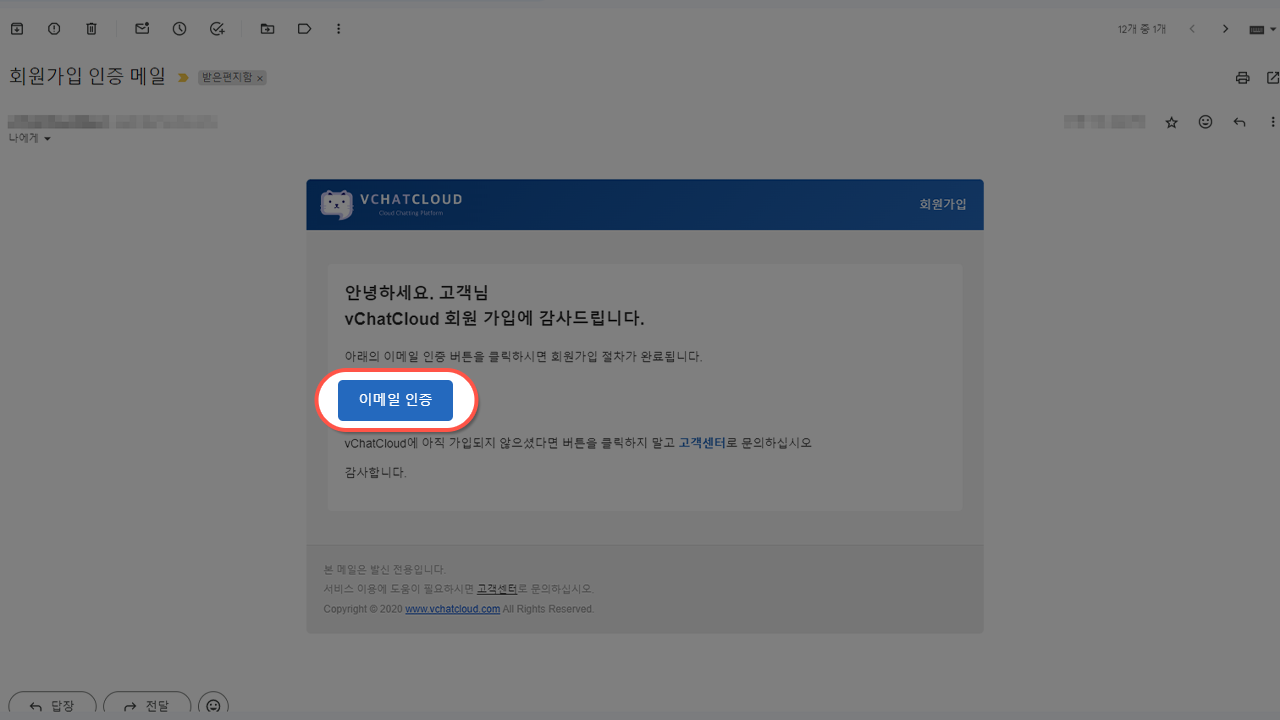
# STEP2. Open a chat room
Click the Login (opens new window) button at the top of vchatCloud's first page.
Please log in with the email and password you registered when registering as a member.
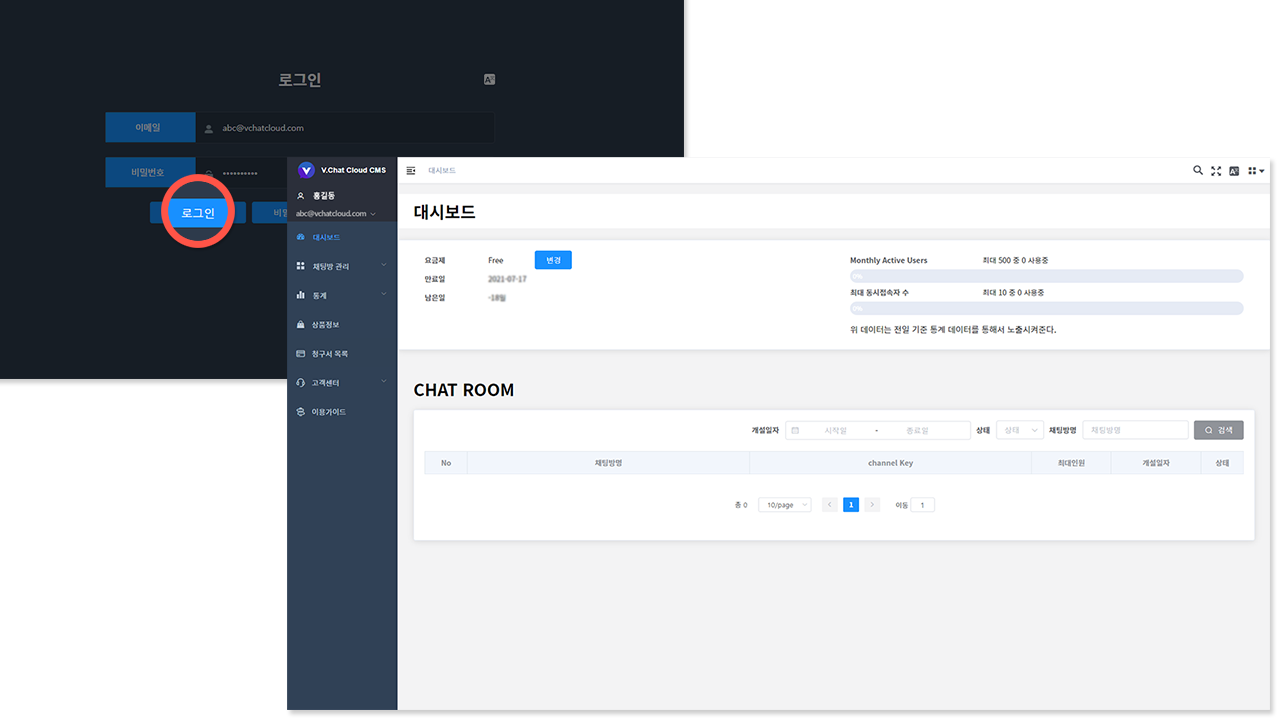
Click Chat Room Management > Chat Room List on the left menu.
Click the Open Chat Room button at the top right.
After entering the chat room title, click the Create button.
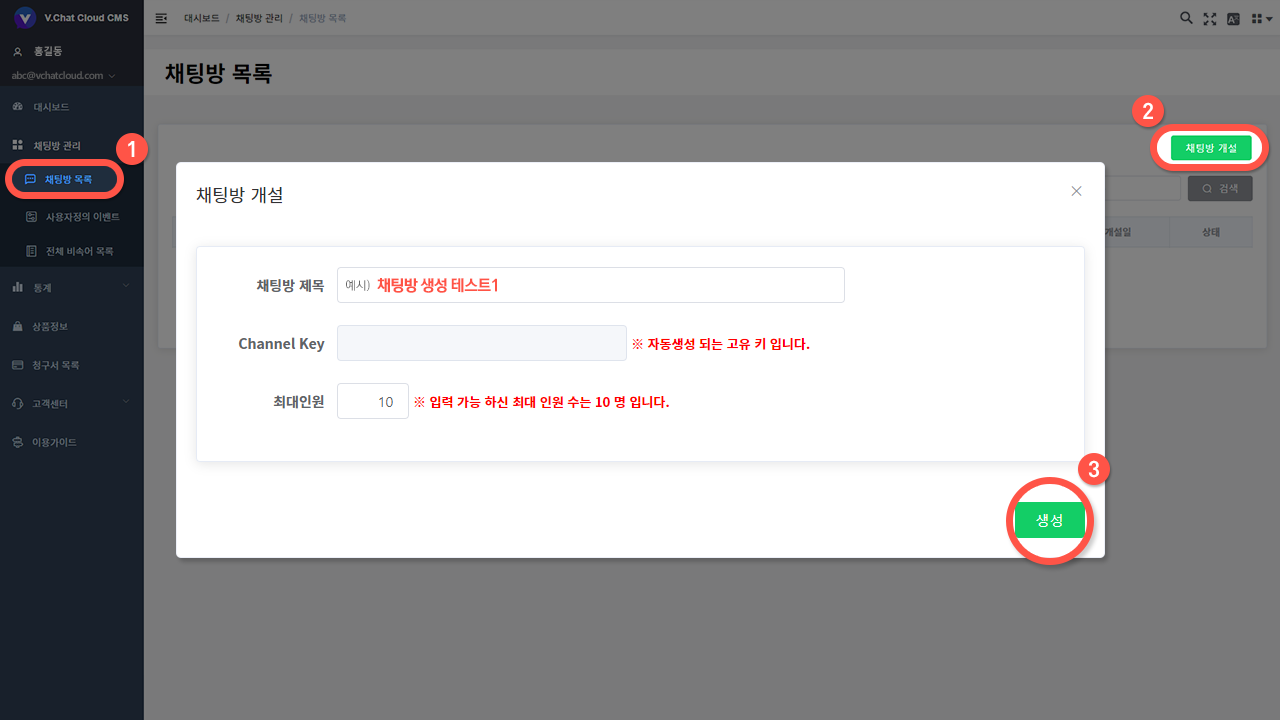
If it was exposed on the chat room list screen, the chat room was created normally.
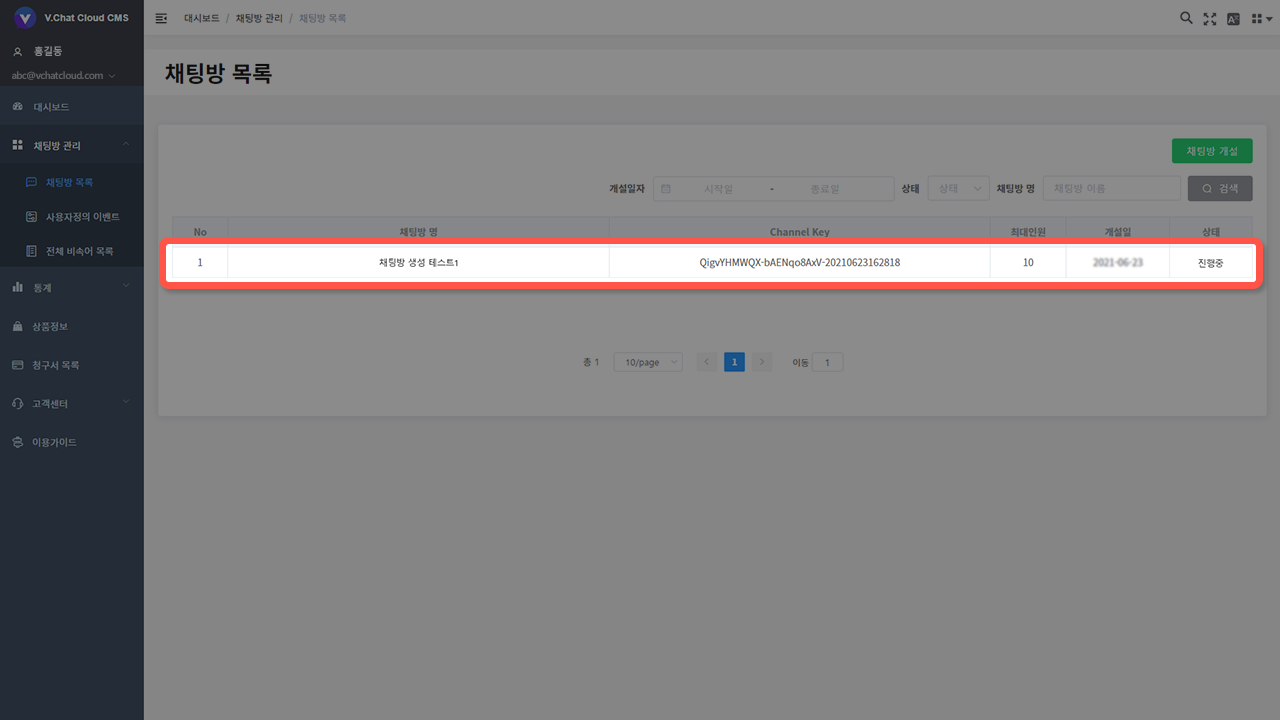
# STEP3. Writing & Running Source Code
# Preparations
To run React UI Kit, you need the following materials.
- Node.js
- npm (or whatever package manager you use)
Please copy the Channel Key
of the chat room you created and write it down separately.
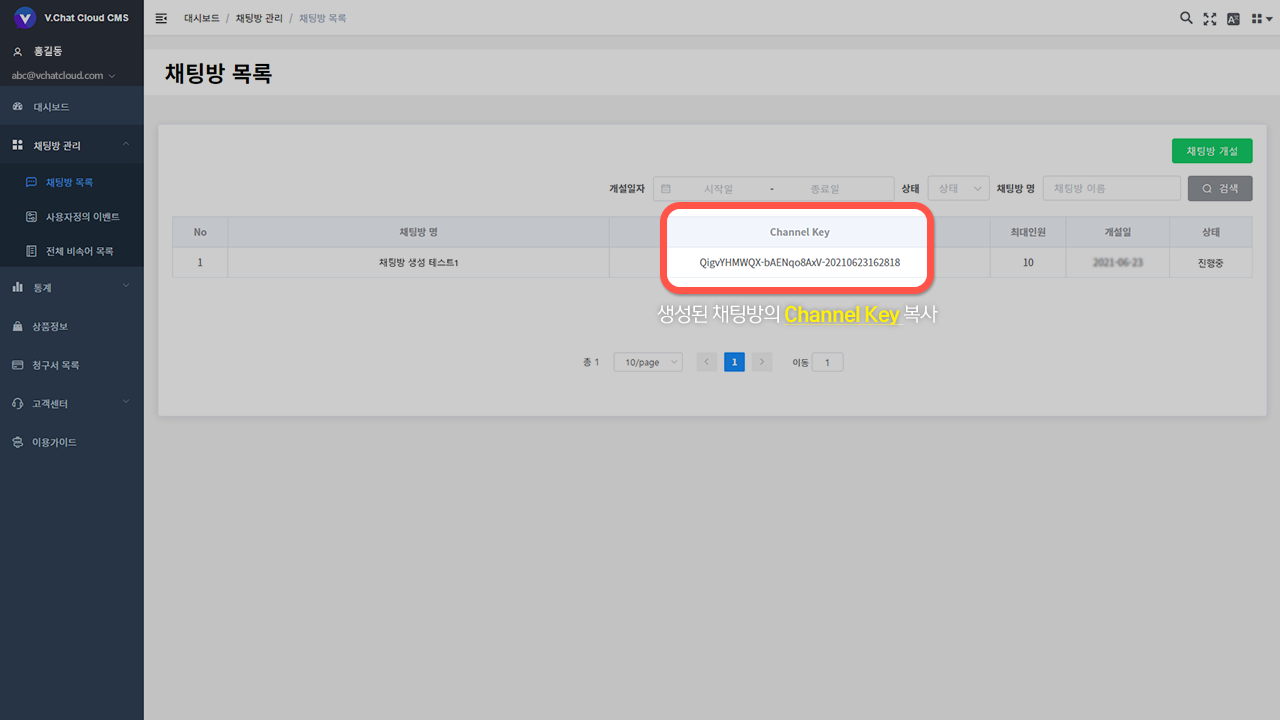
If customization is not necessary, you can simply use the chat room using the iframe method.
- Click the demo screen button on the main homepage, click the menu you want to copy among the various screens, and click the ‘Copy source code’ button to copy it separately.
- Open notepad and paste the copied content. Change
channelKey
in the url part of<iframe src='url' ...></iframe>
in Notepad.- To change
channelKey
, changeVBrhzLZgGG-nAFo5CS7jp-20210802120142
inchannelKey=VBrhzLZgGG-nAFo5CS7jp-20210802120142
to theChannel Key
copied inStep 1
.
- To change
- Save the file as
<file name>.html
(html format). After that, you can access it by running the saved file.
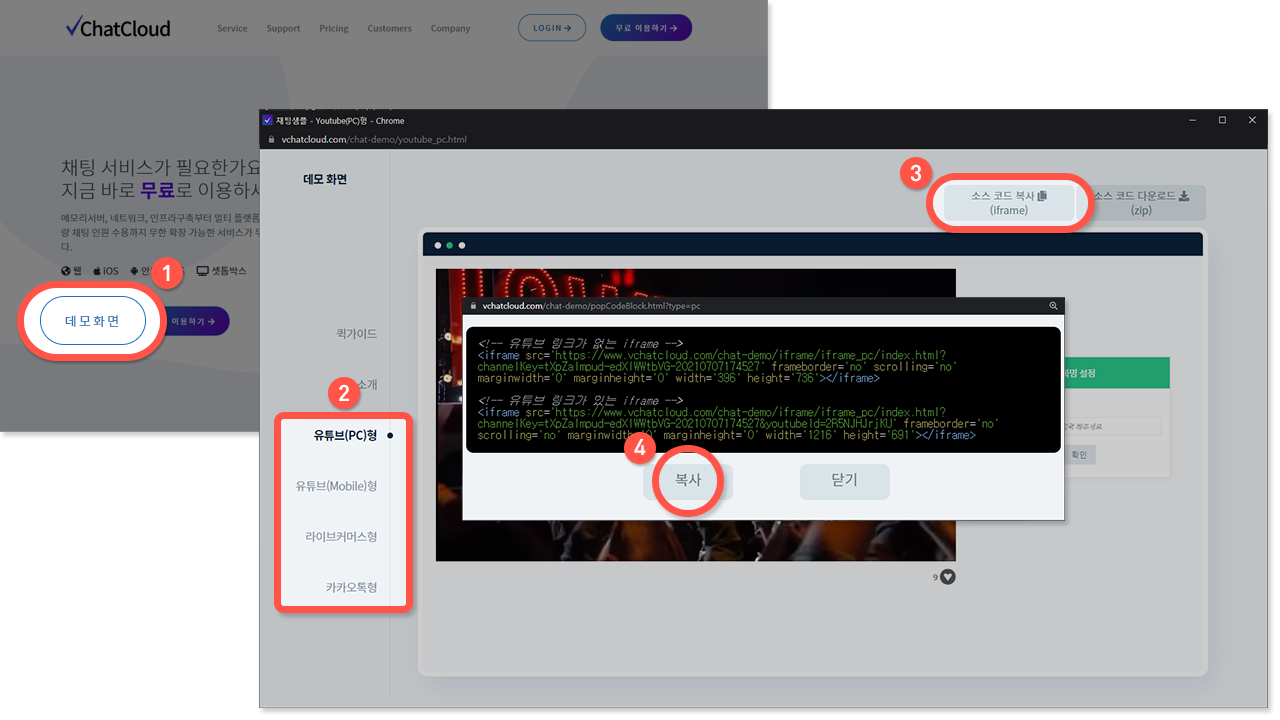
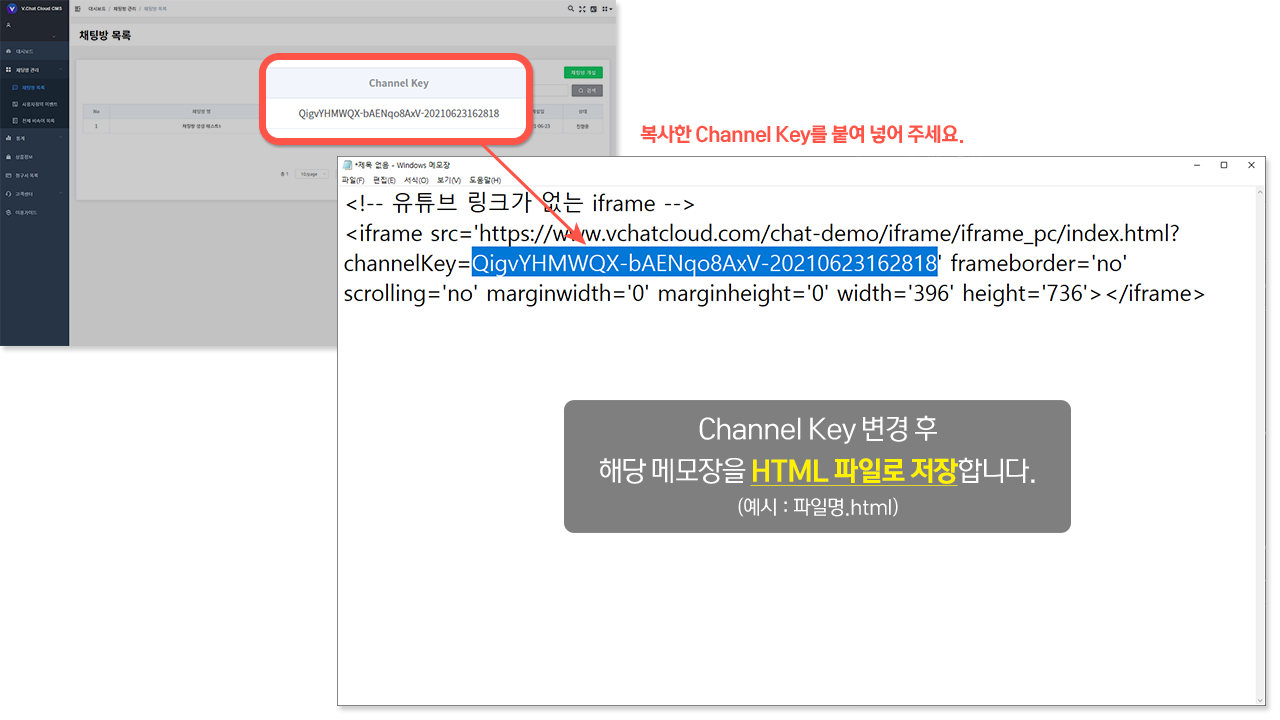
Enter and run the command below in the folder where you want to create the project.
To use TypeScript, you can change the --template
parameter to react-ts
.
npm create vite@latest vchatcloud-react-project -- --template react
After executing the command, select React
from the framework
selection to create a new project.
After that, move to the project folder and run the command below to install @vchatcloud/react-ui-kit
.
cd vchatcloud-react-project
npm install @vchatcloud/react-ui-kit
2
3
Add the following content to index.html
. Write two script
tags between head
.
<head>
<!-- ...your code -->
<script src="https://www.vchatcloud.com/lib/e7lib-latest.min.js"></script>
<script src="https://www.vchatcloud.com/lib/vchatcloud-last.min.js"></script>
</head>
2
3
4
5
After that, enter the following into App.tsx
. However, the necessary values are replaced with new ones.
roomId
: Enter theChannel Key
copied in step 1.clientKey
: Unique key value that does not overlap between usersnickName
: User nickname
import { VChatCloudApp, ChannelUserGrade } from "@vchatcloud/react-ui-kit";
import { FC, useEffect, useRef } from "react";
const App: FC = () => {
const [roomId, clientKey, nickName, grade, userInfo] = [
"YOUR_ROOM_ID",
// You can use any value you want for clientKey, but be careful not to duplicate it between users.
// When logging in with the same clientKey, the previously logged in user will be disconnected.
// Alternatively, you can call and use the `setRandomClientKey` function.
"YOUR_CLIENT_KEY",
"YOUR_NICKNAME",
"user",
{ profile: 1 },
];
const privateContainer = useRef<HTMLDivElement>(null);
// When using private container
useEffect(() => {
if (!privateContainer.current) return;
privateContainer.current.style.display = "none";
const observer = new MutationObserver(() => {
if (!privateContainer.current) return;
privateContainer.current.style.display =
privateContainer.current.children.length === 0 ? "none" : "block";
});
observer.observe(privateContainer.current, { childList: true });
return () => observer.disconnect();
}, []);
return (
<>
<div className="app" style={{ width: "100%", height: "100vh" }}>
<VChatCloudApp
clientKey={clientKey}
email="YOUR_EMAIL" // VChatCloud CMS account ID
grade={grade}
nickName={nickName}
privateContainer=".private-container" // querySelector parameter or HTMLElement
roomId={roomId}
sessionType="parameter"
userInfo={userInfo}
// logoUrl="LOGO_IMG_URL"
// company="YOUR_COMPANY_NAME"
/>
</div>
{/* wrapper for private chat */}
<div
className="app private-container"
ref={privateContainer}
style={{ width: "100%", height: "100vh" }}
/>
</>
);
};
export default App;
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
Run the development server by executing the command below in the terminal.
npm run dev
Then click the address shown below to connect to the development server.
Overview →