# Overview
# Swift
Swift is a programming language developed by Apple and is mostly used to develop apps for Apple operating systems, such as iOS and macOS. Designed with a focus on safety and speed, it allows for more stable code writing through type safety and options. In addition, Swift helps developers develop apps faster and more efficiently by providing intuitive grammar and modern programming capabilities. In addition, Swift's ecosystem is growing continuously, and tools such as Swift Package Manager facilitate the integration of external libraries. In addition, Swift is an open-source project that is developing with contributions from the developer community.
Starting with Swift offers a powerful, modern way to develop apps on the Apple platform. Thanks to its intuitive grammar and powerful performance, many developers prefer Swift.
# Experience chat service
# Enter the chat room
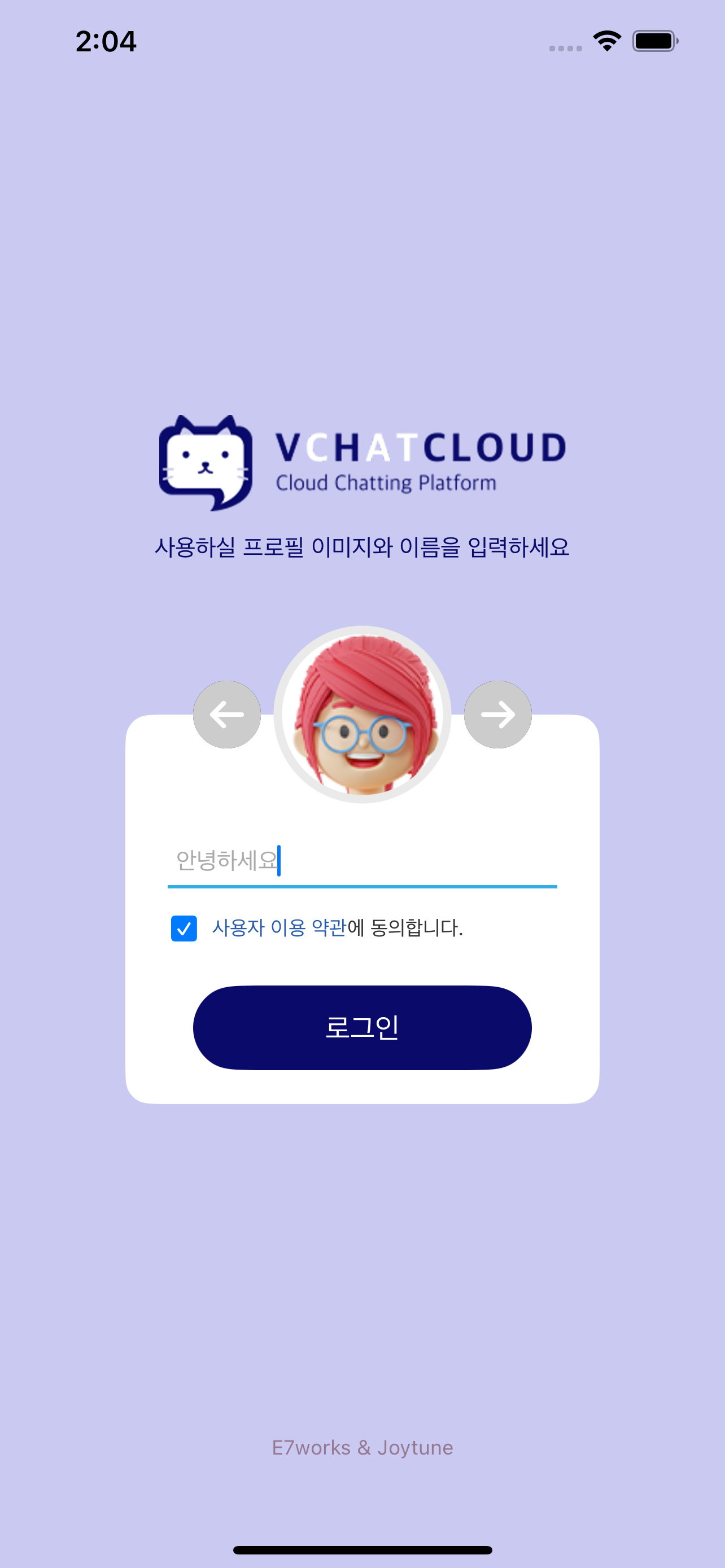
In order to enter the chat room from the demo screen, you must include the channel key generated in the CMS.
If it is before the chat room is opened, please learn how to create a chat room and check the channel key through the QuickStart tab.
After that, enter the chat name and emoji to be used in the chat room, and press the OK button to enter the chat room.
# Chatting
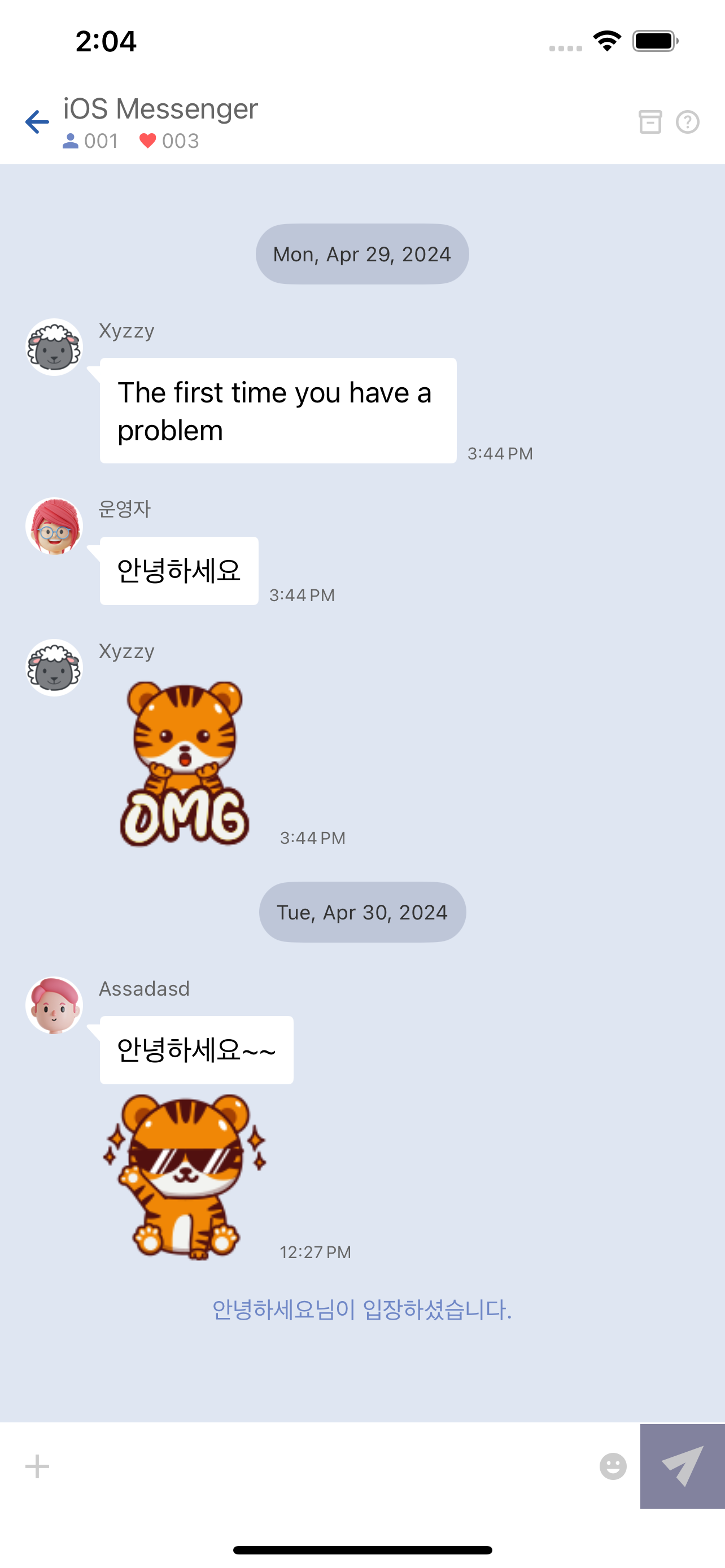
For the chat, type the message you want to send in the text box and press the Send button or the Enter key to send it.
If the message is being sent normally, try entering the chat room with another device or PC.
When entering another user, enter the same chat room by entering the same channel key, and send and receive messages to and from the chat.
# Check the implementation code
# Enter the chat room
import VChatCloudSwiftSDK
// Create a class that complies with the ChannelDelegate protocol.
class MyChannel: ChannelDelegate, ObservableObject {
static var shared: MyChannel = MyChannel()
private init() {}
// ...
func onMessage(_ channelResultModel: ChannelResultModel) {
addMyChatlog(channelResultModel)
}
func onWhisper(_ channelResultModel: ChannelResultModel) {
addMyChatlog(channelResultModel)
}
// ...
}
// Implement functions to run at login
func goLogin() async {
do {
// Channel Key Settings
let channelKey = "YOUR_CHANNEL_KEY" // Change to the channel key issued by CMS.
let channel = try await VChatCloud.shared.connect(chatroomViewModel: chatroomViewModel, userViewModel: userViewModel)
// Register an object that complies with the ChannelDelegate protocol on a Channel object that can communicate with a chat server
// You can process chat events with a delete pattern.
channel.delegate = MyChannel.shared
try await channel.join()
_ = await channel.getClientList()
// You can send messages using the channel.sendMessage method.
channel.sendMessage("Hello VChatCloud!")
} catch {
// Disconnect the connection in the event of an error.
if let channelError = error as? ChannelError {
VChatCloud.shared.disconnect()
} else {
debugPrint("error >>>")
debugPrint(error.localizedDescription)
}
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
# Handling Channel Object Events
You must create a class that complies with the ChannelDelegate
protocol for event processing.
In the example below, we defined the required event processing function by defining the MyChannel
class that complies with ChannelDelegate
.